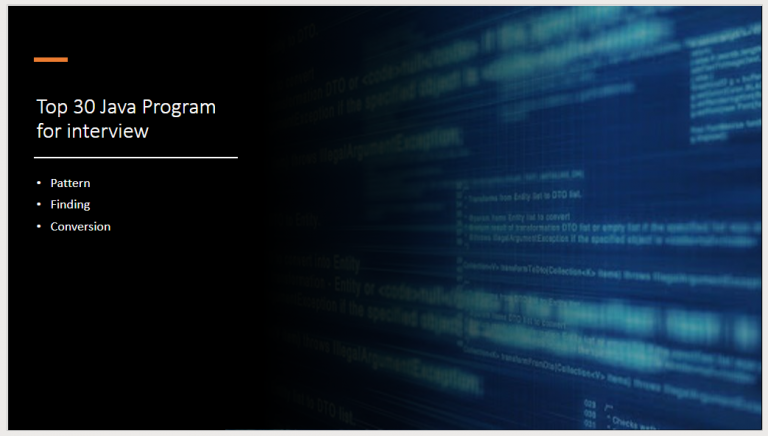
Below are the collection of java program. Hope this Java Program for interview help you to crack the interview of dream company
Hope you also Visited the pervious post also, if not than Please refer that also
This Articles Contents
Write a java program to Convert Binary to Decimal
package com.onurdesk.basic.interview.programs;
import java.io.*;
class BinaryToDecimal
{
public static void main(String[] args) throws Exception
{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.print("Enter Binary no. to convert in Decimal : ");
String number = br.readLine();
/*
to convert Binary number to decimal number use,
int parseInt method of Integer wrapper class.
Pass 2 as redix second argument.
*/
int decimalNumber = Integer.parseInt(number, 2);
System.out.println("Binary number converted to decimal number");
System.out.println("Decimal number is : " + decimalNumber);
}
}

Write a java program to Convert Binary to Octal
import java.io.*;
class BinaryToOctal
{
public static void main(String[] args) throws Exception
{
String num = null;
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
System.out.print("Enter binary number : ");
num = br.readLine();
int dec = Integer.parseInt(num, 2);
String oct = Integer.toOctalString(dec);
System.out.println("Binary " + num + " in Octal is " + oct);
}
}

Write a java program to Convert Decimal to Binary
package com.onurdesk.basic.interview.programs;
import java.util.Scanner;
class DecimalToBinary {
public String toBinary(int n) {
if (n == 0) {
return "0";
}
String binary = "";
while (n > 0) {
int rem = n % 2;
binary = rem + binary;
n = n / 2;
}
return binary;
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number: ");
int decimal = scanner.nextInt();
DecimalToBinary decimalToBinary = new DecimalToBinary();
String binary = decimalToBinary.toBinary(decimal);
System.out.println("The binary representation is " + binary);
}
}

Write a java program to Convert Decimal to Binary
import java.util.Scanner;
class DecimalToBinary
{
public String toBinary(int n)
{
if (n == 0)
{
return "0";
// Java Program for interview
}
String binary = "";
while (n > 0)
{
int rem = n % 2;
binary = rem + binary;
n = n / 2;
}
return binary;
}
public static void main(String[] args)
{
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number: ");
int decimal = scanner.nextInt();
DecimalToBinary decimalToBinary = new DecimalToBinary();
String binary = decimalToBinary.toBinary(decimal);
System.out.println("The binary representation is " + binary);
}
}

Write a java program to Find Fraction Addition in java
package com.onurdesk.basic.interview.programs;
import java.util.*;
class FractionAdding {
public static void main(String args[]) {
float a, b, c, d;
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a : ");
a = scanner.nextFloat();
System.out.print("Enter b : ");
b = scanner.nextFloat();
System.out.print("Enter c : ");
c = scanner.nextFloat();
System.out.print("Enter d : ");
d = scanner.nextFloat();
// Java Program for interview
// fraction addition formula ((a*d)+(b*c))/(b*d)
System.out.print("Fraction Addition : [( " + a + " * " + d + " )+( " + b + " * " + c + " ) / ( " + b + " * " + d
+ " )] = " + (((a * d) + (b * c)) / (b * d)));
}
}

Write a java program to Find Fraction Subtraction
package com.onurdesk.basic.interview.programs;
import java.util.*;
class FractionSubtraction {
public static void main(String args[]) {
float a, b, c, d;
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a : ");
a = scanner.nextFloat();
System.out.print("Enter b : ");
b = scanner.nextFloat();
System.out.print("Enter c : ");
c = scanner.nextFloat();
System.out.print("Enter d : ");
d = scanner.nextFloat();
// fraction addition formula ((a*d)-(b*c))/(b*d)
System.out.print("Fraction subtraction : [( " + a + " * " + d + " )-( " + b + " * " + c + " ) / ( " + b + " * "
+ d + " )] = " + (((a * d) - (b * c)) / (b * d)));
// Java Program for interview
}
}

Write a java program to Find GCDLCM.
import java.util.Scanner;
class GCDLCM
{
public static void main(String args[])
{
int x, y;
Scanner sc = new Scanner(System.in);
System.out.println("Enter the two numbers: ");
x = sc.nextInt();
y = sc.nextInt();
System.out.println("GCD of two numbers is : " + gcd(x, y));
System.out.println("LCM of two numbers is : " + lcm(x, y));
}
static int gcd(int x, int y)
{
int r = 0, a, b;
a = (x > y) ? x : y; // a is greater number
b = (x < y) ? x : y; // b is smaller number
r = b;
while (a % b != 0)
{
r = a % b;
a = b;
b = r;
}
return r;
}
static int lcm(int x, int y)
{
int a;
a = (x > y) ? x : y; // a is greater number
while (true)
{
if (a % x == 0 && a % y == 0)
{
return a;
}
++a;
}
}
}

Write a java program to Find Harmonic Series.
class HarmonicSeries
{
public static void main(String args[])
{
int num, i = 1;
double rst = 0.0;
Scanner in = new Scanner(System.in);
System.out.println("Enter the number for length of series");
num = in.nextInt();
// Java Program for interview
while (i <= num)
{
System.out.print("1/" + i + " +");
rst = rst + (double) 1 / i;
i++;
}
System.out.println("\n\nSum of Harmonic Series is " + rst);
}
}

Write a java program to Create a Multiplication Table
package com.onurdesk.basic.interview.programs;
import java.util.Scanner;
class MultiplicationTable {
public static void main(String args[]) {
int n, c;
System.out.println("Enter an integer to print it's multiplication table");
Scanner in = new Scanner(System.in);
n = in.nextInt();
System.out.println("Multiplication table of " + n + " is :-");
for (c = 1; c <= 10; c++) {
System.out.println(n + "*" + c + " = " + (n * c));
}
}
}

Write java program for Alphabet Pattern in Java
package com.onurdesk.basic.interview.programs;
import java.util.*;
class AlphabetPattern
{
public static void main(String[] arg)
{
int line, row, col;
char ch = 'A';
Scanner scanner = new Scanner(System.in);
System.out.print("Enter number of lines : ");
line = scanner.nextInt();
for (row = 1; row <= line; row++)
{
for (col = 1; col <= row; col++)
{
System.out.print("" + ch);
}
System.out.println();
ch++;
}
}
}
Write a java program Binary Pattern
class BinaryPattern
{
public static void main(String s[])
{
int i, j;
int count = 1;
for (i = 1; i <= 5; i++)
{
for (j = 1; j <= i; j++)
{
System.out.format("%d", count++ % 2);
if (j == i && i != 5)
System.out.println("");
}
if (i % 2 == 0)
count = 1;
else
count = 0;
}
}
}

Write a program for Christmas tree pattern java.
package com.onurdesk.basic.interview.programs;
class ChristmasTree {
public static final int segments = 4;
public static final int height = 4;
public static void main(String[] args) {
makeTree();
}
public static void makeTree() {
int maxStars = 2 * height + 2 * segments - 3;
String maxStr = "";
for (int l = 0; l < maxStars; l++) {
maxStr += " ";
}
for (int i = 1; i <= segments; i++) {
for (int line = 1; line <= height; line++) {
String starStr = "";
for (int j = 1; j <= 2 * line + 2 * i - 3; j++) {
starStr += "*";
}
for (int space = 0; space <= maxStars - (height + line + i); space++) {
starStr = " " + starStr;
}
System.out.println(starStr);
}
}
for (int i = 0; i <= maxStars / 2; i++) {
System.out.print(" ");
}
System.out.println(" " + "*" + " ");
for (int i = 0; i <= maxStars / 2; i++) {
System.out.print(" ");
}
System.out.println(" " + "*" + " ");
for (int i = 0; i <= maxStars / 2 - 3; i++) {
System.out.print(" ");
}
System.out.println(" " + "*******");
}
}

Write a program Christmas tree pattern in Java
class ChristmasTreePattern
{
public static void main(String[] arg)
{
drawChristmasTree(4);
}
private static void drawChristmasTree(int n)
{
for (int i = 0; i < n; i++)
{
triangle(i + 1, n);
}
}
private static void triangle(int n, int max)
{
for (int i = 0; i < n; i++)
{
for (int j = 0; j < max - i - 1; j++)
{
System.out.print(" ");
}
for (int j = 0; j < i * 2 + 1; j++)
{
System.out.print("X");
}
System.out.println("");
}
}
}

Write a program for Floyd Triangle in java
import java.util.Scanner;
class FloydTriangle
{
public static void main(String args[])
{
int n, num = 1, c, d;
Scanner in = new Scanner(System.in);
System.out.print("Enter the number of rows of floyd's triangle : ");
n = in.nextInt();
System.out.println("Floyd's triangle :-");
for (c = 1; c <= n; c++)
{
for (d = 1; d <= c; d++)
{
System.out.print(num + " ");
num++;
}
System.out.println();
}
}
}

Write a program for the below number pattern in java
1
12
123
1234
12345
package com.onurdesk.basic.interview.programs;
class NumberPat1 {
public static void main(String arg[]) {
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= i; j++) {
System.out.print(j);
}
System.out.println();
}
}
}

Write a program for the below number pattern in java
54321
5432
543
54
5
class NumberPat2
{
public static void main(String arg[])
{
for (int i = 1; i <= 5; i++)
{
for (int j = 5; j >= i; j--)
{
System.out.print(j);
}
System.out.println();
}
}
}

Write a program for the below number pattern in java
12345
1234
123
12
1
package com.onurdesk.numberpattern.program;
class NumberPat3 {
public static void main(String arg[]) {
for (int i = 1, r = 5; i <= 5; i++, r--) {
for (int j = 1; j <= r; j++) {
System.out.print(j);
}
System.out.println();
}
System.out.println("Thanks for visiting https://onurdesk.com");
}
}

Write a program for the below number pattern in java
1
12
123
1234
12345
1234
123
12
1
package com.onurdesk.numberpattern.program;
/**
* @author Onurdesk
*
* Thanks for visiting https://onurdesk.com like us on facebook :
* https://facebook.com/onurdesk
*/
class NumberPat4 {
public static void main(String arg[]) {
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= i; j++) {
System.out.print(j);
}
System.out.println();
}
for (int i = 1, r = 5 - 1; i <= 5 - 1; i++, r--) {
for (int j = 1; j <= r; j++) {
System.out.print(j);
}
System.out.println();
}
System.out.println("Thanks for visiting https://onurdesk.com");
}
}
Write a program for the below number pattern in java.
12345
1234
123
12
1
12
123
1234
12345
package com.onurdesk.numberpattern.program;
class NumberPat5
{
/**
* @author Onurdesk
*
* Thanks for visiting https://onurdesk.com like us on facebook :
* https://facebook.com/onurdesk
*/
public static void main(String arg[])
{
for (int i = 1, r = 5; i <= 5; i++, r--)
{
for (int j = 1; j <= r; j++)
{
System.out.print(j);
}
System.out.println();
}
for (int i = 1; i <= 5; i++)
{
for (int j = 1; j <= i; j++)
{
System.out.print(j);
}
System.out.println();
}
System.out.println("Thanks for visiting https://onurdesk.com");
}
}

.
Write a program for the below number pattern in java.
1
22
333
4444
55555
package com.onurdesk.numberpattern.program;
class NumberPat6
{
/**
* @author Onurdesk
*
* Thanks for visiting https://onurdesk.com like us on facebook :
* https://facebook.com/onurdesk
*/
public static void main(String arg[])
{
for(int i=1;i<=5;i++)
{
for(int j=1;j<=i;j++)
{
System.out.print(i);
}
System.out.println();
}
System.out.println("Thanks for visiting https://onurdesk.com");
}
}
Write a program for the below number pattern in java.
1
23
456
7890
12345
package com.onurdesk.numberpattern.program;
class NumberPat7
{
/**
* @author Onurdesk
*
* Thanks for visiting https://onurdesk.com like us on facebook :
* https://facebook.com/onurdesk
*/
public static void main(String arg[])
{
int t = 1;
for (int i = 1; i <= 5; i++)
{
for (int j = 1; j <= i; j++)
{
if (t == 10) {
t = 0;
}
System.out.print(t++);
}
System.out.println();
}
System.out.println("Thanks for visiting https://onurdesk.com");
}
}

Write a program for the below number pattern in java.
5
11111
0000
111
00
1
package com.onurdesk.numberpattern.program;
import java.util.Scanner;
class Pattern
{
/**
* @author Onurdesk
*
* Thanks for visiting https://onurdesk.com like us on facebook :
* https://facebook.com/onurdesk
*/
public static void main(String args[])
{
int n, i, j;
Scanner sc = new Scanner(System.in);
System.out.println("Enter the number of rows ");
n = sc.nextInt();
for (i = 1; i <= n; i++)
{
for (j = i; j <= n; j++)
{
if (i % 2 == 0)
System.out.print("0");
else
System.out.print("1");
}
System.out.println();
}
System.out.println("Thanks for visiting https://onurdesk.com");
}
}

Write a program for the below number pattern in java.
1
1 2 1
1 2 3 2 1
1 2 3 4 3 2 1
1 2 3 4 5 4 3 2 1
package com.onurdesk.numberpattern.program;
class PrintPattern
{
/**
* @author Onurdesk
*
* Thanks for visiting https://onurdesk.com like us on facebook :
* https://facebook.com/onurdesk
*/
public static void main(String args[])
{
int n = 5;
for (int i = 1; i <= n; i++)
{
int j = n - i;
while (j > 0)
{
System.out.print(" ");
j--;
}
j = 1;
while (j <= i)
{
System.out.print(" " + j);
j++;
}
j = i - 1;
while (j > 0)
{
System.out.print(" " + j);
j--;
}
System.out.println( );
j = n - i;
while (j > 0)
{
System.out.print(" ");
j--;
}
System.out.println();
}
System.out.println("Thanks for visiting https://onurdesk.com");
}
}
Write a program for the below number pattern in java.
1
121
12321
1234321
123454321
12345654321
1234567654321
package com.onurdesk.numberpattern.program;
import java.util.*;
class Patternpat2
/**
* @author Onurdesk
*
* Thanks for visiting https://onurdesk.com like us on facebook :
* https://facebook.com/onurdesk
*/
{ public static void main(String[] args){
int i, j, k = 1, l, n;
Scanner sc = new Scanner(System.in);
System.out.println("Enter the number of levels of pattern");
n = sc.nextInt();
System.out.println("\nPattern is : \n");
for (i = 1; i <= n; i++)
{
l = i;
for (j = 1; j <= k; j++)
{
if (j >= i + 1)
{
System.out.print(--l);
}
else
{
System.out.print(j);
}
}
k = k + 2;
System.out.println(" ");
}
System.out.println("Thanks for visiting https://onurdesk.com");
}
}
Write a program for the below star pattern in java.
****
***
**
*
package com.onurdesk.numberpattern.program;
class StarPattern1
{
/**
* @author Onurdesk
*
* Thanks for visiting https://onurdesk.com like us on facebook :
* https://facebook.com/onurdesk
*/
public static void main(String arg[])
{
for (int i = 1; i <= 5; i++)
{
for (int j = i; j <= 5; j++)
{
System.out.print("*");
}
System.out.println();
}
System.out.println("Thanks for visiting https://onurdesk.com");
}
}

Write a program for the below star pattern in java.
*
**
***
****
*****
package com.onurdesk.numberpattern.program;
class StarPattern2
{
/**
* @author Onurdesk
*
* Thanks for visiting https://onurdesk.com like us on facebook :
* https://facebook.com/onurdesk
*/
public static void main(String arg[])
{
for (int i = 1; i <= 5; i++)
{
for (int j = 1; j <= i; j++)
{
System.out.print("*");
}
System.out.println();
}
System.out.println("Thanks for visiting https://onurdesk.com");
}
}

Write a program for the below star pattern in java.
*****
****
***
**
*
*
**
***
****
*****
package com.onurdesk.numberpattern.program;
class StarPattern3
{
/**
* @author Onurdesk
*
* Thanks for visiting https://onurdesk.com like us on facebook :
* https://facebook.com/onurdesk
*/
public static void main(String arg[])
{
for (int i = 1; i <= 5; i++)
{
for (int j = i; j <= 5; j++)
{
System.out.print("*");
}
System.out.println();
}
for (int i = 1; i <= 5; i++)
{
for (int j = 1; j <= i; j++)
{
System.out.print("*");
}
System.out.println();
}
System.out.println("Thanks for visiting https://onurdesk.com");
}
}

Write a program for the below star pattern in java.
* *
** **
*** ***
**** ****
***** *****
************
package com.onurdesk.numberpattern.program;
class StarPattern4
{
public static void main(String arg[])
{
int num = 12;
int f = 2;
int g = num - 1;
for (int i = 1; i <= (num / 2); i++)
{
for (int j = 1; j <= num; j++)
{
if (j >= f && j <= g)
{
System.out.print(" ");
}
else
{
System.out.print("*");
}
}
f = f + 1;
g = g - 1;
System.out.println();
}
}
}

Write a program for the below star pattern in java.
*
**
***
****
*****
*****
****
***
**
*
package com.onurdesk.numberpattern.program;
class StarPattern5
{
public static void main(String arg[])
{
for (int i = 1; i <= 5; i++)
{
for (int j = 1; j <= i; j++)
{
System.out.print("*");
}
System.out.println();
}
for (int i = 1; i <= 5; i++)
{
for (int j = i; j <= 5; j++)
{
System.out.print("*");
}
System.out.println();
}
}
}

Write a program for the below star pattern in java.
6
*
***
*****
*******
*********
***********
package com.onurdesk.numberpattern.program;
import java.io.*;
class Triangle
{
public static void main(String arg[])
{
InputStreamReader istream = new InputStreamReader(System.in);
BufferedReader read = new BufferedReader(istream);
System.out.println("Enter Triangle Size : ");
int num = 0;
try
{
num = Integer.parseInt(read.readLine());
}
catch (Exception Number)
{
System.out.println("Invalid Number!");
}
for (int i = 1; i <= num; i++)
{
for (int j = 1; j < num - (i - 1); j++)
{
System.out.print(" ");
}
for (int k = 1; k <= i; k++)
{
System.out.print("*");
for (int k1 = 1; k1 < k; k1 += k)
{
System.out.print("*");
}
}
System.out.println();
}
}
}

[…] creating a series of basic interview program in Python, We learn step by step. You can also visit Java program if you are looking for […]